Regarding the class material and the recent homework, I felt like I needed to better understand when it is more appropriate to use each one. Luckily I found a guide for every design pattern at tutorials point, which I used as reference for last week’s homework.
Starting off with the Strategy Pattern, the idea is to separate the program functionality among different algorithms/objects so that the program can perform any given task independently from the context of another function. This gives the application a lot of flexibility and re-usability when choosing what to execute at runtime, as different strategies can be easily added or modified without changing the object’s core code.
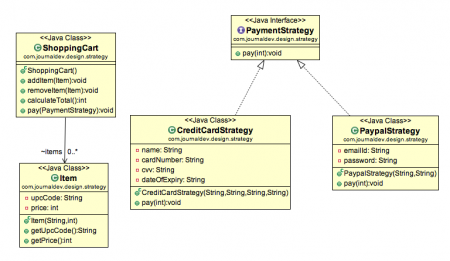
Per the above image, the Strategy interface defines an action and the concrete strategy classes implement said Strategy interface. Both concrete classes implement the pay() function yet they define it differently without breaking the code while doing so.
Another design pattern used was the Singleton Pattern, which ensures a class has only one instance of an object by handling its construction and global access point by itself. This is particularly useful when you want to limit the instantiation of a class to a single object, because in some cases multiple instances being active at the same time can cause weird bugs. Therefore, we use the singleton pattern to regulate the global state of an application. Though by doing so, we are relying on a monolithic design structure that WILL limit variations in its behavior, contrary to the previously mentioned Strategy Pattern that organizes and manages everything in a more structured yet flexible way.
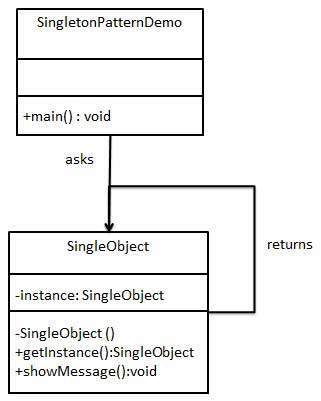
Quite simply, a Singleton class has its constructor as private and has a static instance of itself while the SingleObject class provides a static method to get its static instance to global.
Last but not least is the Factory Pattern, they are similar to strategy pattern in the sense that it separates functionality between different objects yet it’s used when the construction of a particular object needs a dependency that you do not want for the actual class, i.e. adding a rubber duck behavior to the child of superclass duck. This allows our code to create different objects without rewriting/modifying preexisting superclasses since everything is modular in its construction.
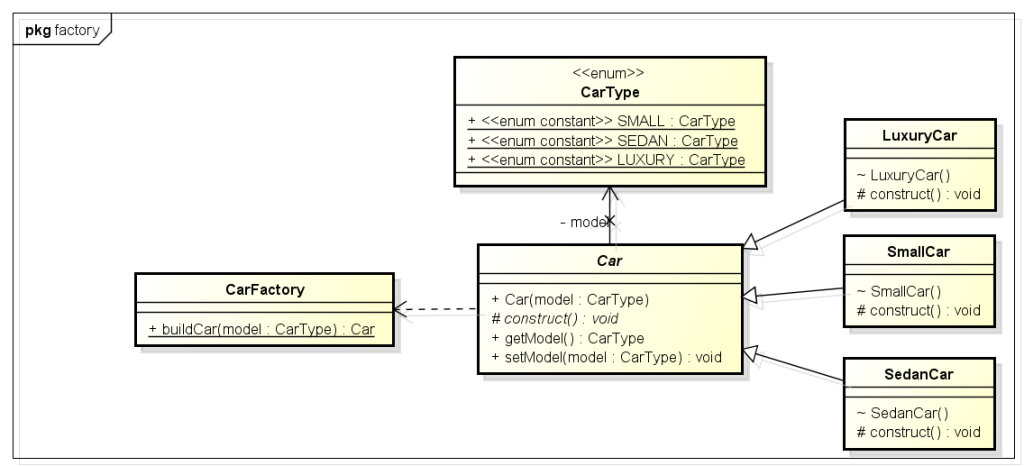
In this example, notice how an object car can be constructed as a small,sedan,luxury car without losing functionality from the base car code. This follows the principle of loose coupling between classes which makes the code more flexible and less fragile.
Overall I think after what I learned this week I am more proficient at detecting design smells and how to better implement design structures based on any given situation. With these design patterns ingrained in my toolkit, I’ve come to understand the value of adaptability (malleability?) in software architecture. Their applications extend beyond just classwork; they serve as guiding principles, enabling the creation of systems capable of evolving alongside dynamic project requirements. This deeper insight into design patterns has illuminated the art of balancing structure and flexibility, paving the way for more elegant, scalable, and resilient software solutions. I’m now thrilled by the prospect of employing these learned strategies to navigate the intricate landscapes of programming, fostering innovation, and robustness in my future projects.
REFERENCES
Design Patterns – Tutorials Point
https://www.tutorialspoint.com/design_pattern/singleton_pattern.htm
A Beginner’s Guide to the Strategy Design Pattern – FreeCodeCamp
https://www.freecodecamp.org/news/a-beginners-guide-to-the-strategy-design-pattern/